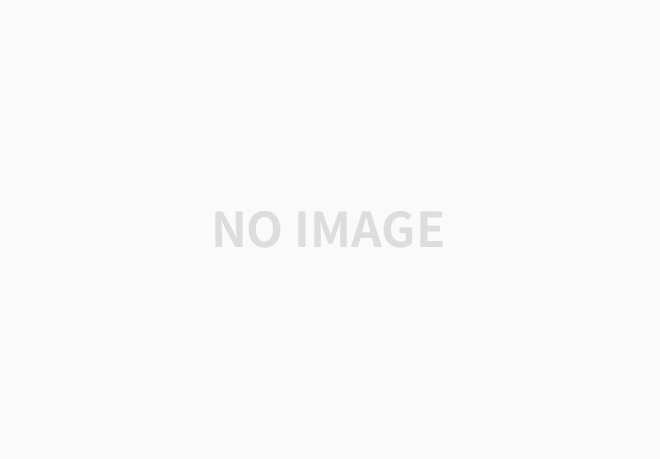
๐ฐ FastAPI ๊ณต์๋ฌธ์๋ฅผ ๋ณด๋ฉด์ ๊ฐ์ธ์ ์ผ๋ก ์ ๋ฆฌํ ๊ธ ์
๋๋ค.
์๋ต์ํ
HTTP์์๋ ์๋ต์ํ์ ๋ฐ๋ผ ์ํ์ฝ๋๋ฅผ ๋ฐํํ๋ค.
FastAPI์์๋ status_code ๋งค๊ฐ๋ณ์๋ฅผ ์ฌ์ฉํ์ฌ ์๋ต์ ๋ํ HTTP ์ํ ์ฝ๋๋ฅผ ์ ์ธํ ์ ์๋ค.
๊ทธ๋ฆฌ๊ณ ๋ชจ๋ ์ํ์ฝ๋๋ฅผ ์ธ์ธ ํ์ ์์ด fastapi์ ํ์ ํด๋์ค status์์ ๋ณ์๋ฅผ ์ฐพ์ ์ฌ์ฉํ ์ ์๋ค.
from fastapi import FastAPI, status
app = FastAPI()
@app.post("/items/", status_code=status.HTTP_201_CREATED)
async def create_item(name: str):
return {"name": name}
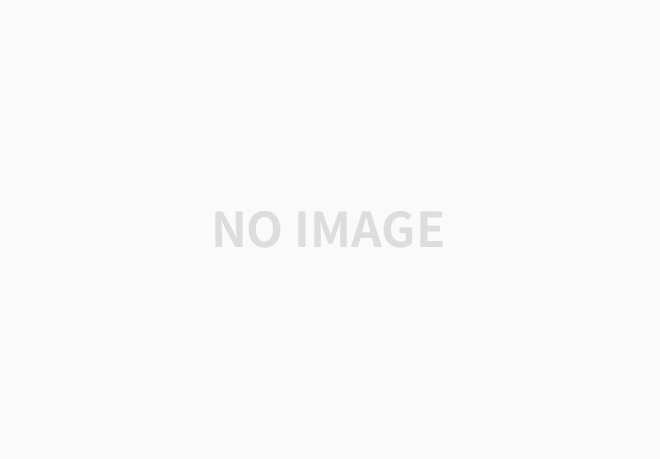
Form
Form ํด๋์ค๋ฅผ ์ฌ์ฉํ์ฌ Form ํ๋์ ๋์์ ์ ์ดํ๊ณ ์ ํจ์ฑ ๊ฒ์ฌ๋ฅผ ์ํํ ์ ์๋ค.
์ฃผ์ ๋งค๊ฐ๋ณ์๋ ๋ค์๊ณผ ๊ฐ๋ค.
- default : ํ๋์ ๊ธฐ๋ณธ๊ฐ์ ์ค์ ํฉ๋๋ค. ์ฌ์ฉ์๊ฐ ํด๋น ํ๋์ ๊ฐ์ ์ ๋ ฅํ์ง ์์ ๊ฒฝ์ฐ ์ด ๊ฐ์ด ์ฌ์ฉ๋ฉ๋๋ค.
- title : ํ๋์ ์ ๋ชฉ์ด๋ ๋ผ๋ฒจ์ ์ค์ ํฉ๋๋ค. ์ด ๊ฐ์ ํผ ์์์ ๋ ์ด๋ธ๋ก ํ์๋ฉ๋๋ค.
- description : ํ๋์ ๋ํ ์ถ๊ฐ ์ค๋ช ์ ์ค์ ํฉ๋๋ค. ์ด ๊ฐ์ ํผ ์์์ ์ค๋ช ์ผ๋ก ํ์๋ฉ๋๋ค.
- min_length ๋ฐ max_length : ์ ๋ ฅ ๊ฐ์ ์ต์ ๊ธธ์ด์ ์ต๋ ๊ธธ์ด๋ฅผ ์ค์ ํฉ๋๋ค.
- regex : ์ ๋ ฅ ๊ฐ์ ํ์์ ์ ๊ท์์ผ๋ก ์ ํํฉ๋๋ค. ์ ๋ ฅ ๊ฐ์ ์ด ์ ๊ท์๊ณผ ์ผ์นํด์ผ ํฉ๋๋ค.
- deprecated : ํ๋๊ฐ ์ฌ์ฉ๋์ง ์๋๋ก ํ์ํ ์ง ์ฌ๋ถ๋ฅผ ์ค์ ํฉ๋๋ค. ๊ธฐ๋ณธ๊ฐ์ False์ ๋๋ค.
- alias : ํ๋์ ๋ณ์นญ์ ์ค์ ํฉ๋๋ค. ์ด ๊ฐ์ ์ฌ์ฉํ๋ฉด ํ๋์ ์ด๋ฆ์ ๋ค๋ฅธ ์ด๋ฆ์ผ๋ก ์ฌ์ฉํ ์ ์์ต๋๋ค.
- ge์ le : ์ซ์ ํ์์ ํ๋์์ ์ฌ์ฉ๋๋ฉฐ, ์ต์๊ฐ (ge)๊ณผ ์ต๋๊ฐ (le)์ ์ค์ ํฉ๋๋ค.
from typing import Union
from fastapi import FastAPI, Form
from fastapi.responses import HTMLResponse
from pydantic import BaseModel, EmailStr
app = FastAPI()
class UserIn(BaseModel):
username: str
password: str
email: EmailStr
nickname: str
class UserOut(BaseModel):
username: str
email: EmailStr
nickname: str
forms = {
'username': {'min_length': 5, 'max_length': 15, 'title': 'Username', 'description': 'ID'},
'password': {'min_length': 8, 'max_length': 15, 'title': 'Password', 'description': 'ํจ์ค์๋'},
'email': {'min_length': 5, 'max_length': 20, 'title': 'Email', 'description': '์ด๋ฉ์ผ'},
'nickname': {'min_length': 2, 'max_length': 8, 'title': 'Nick_Name', 'description': '๋๋ค์'}
}
@app.post("/user/", response_model=UserOut)
async def createUser(
username: str = Form(..., **forms['username']),
password: str = Form(..., **forms['password']),
email: str = Form(..., **forms['email']),
nickname: str = Form(..., **forms['nickname'])
):
user = UserIn(username=username, password=password,
email=email, nickname=nickname)
return user
context = """
<body>
<form method="post" action="/user">
ID : <input type="text" name="username"><br>
PW : <input type="password" name="password"><br>
Email : <input type="email" name="email"><br>
Nickname : <input type="text" name="nickname"><br>
<input type='submit'>
</form>
</body>
"""
@app.get('/')
async def main():
return HTMLResponse(context)
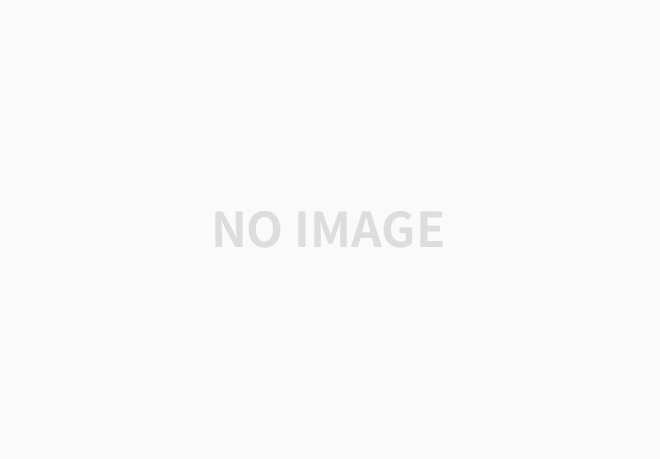
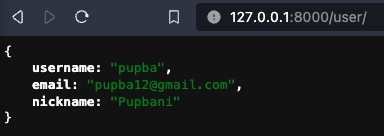
File
File์ ์ฌ์ฉํ์ฌ ํด๋ผ์ด์ธํธ๊ฐ ์ ๋ก๋ํ ํ์ผ์ ์ ์ํ ์ ์๋ค.
File ๋งค๊ฐ๋ณ์๋ฅผ ์ฌ์ฉํ์ฌ ํ์ผ์ ๋ฐ์ ์ ์๋ค.(File์ Form์ ์์ํ ์์ ํด๋์ค์ด๋ค.)
from fastapi import FastAPI, File
app = FastAPI()
@app.post("/file/")
async def createFile(file: bytes = File()):
return {"file_size": len(file)}
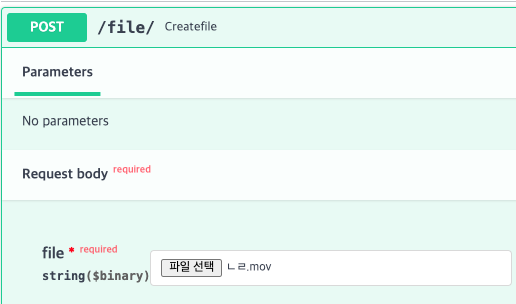
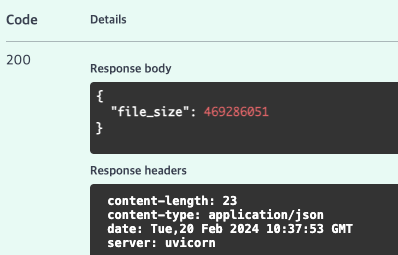
File ๋์ UploadFile์ ์ฌ์ฉํ ์ ์๋ค.
- ์คํ ํ์ผ ์ฌ์ฉ(์ต๋ ํฌ๊ธฐ ์ ํ๊น์ง๋ง ๋ฉ๋ชจ๋ฆฌ์ ์ ์ฅ๋๊ณ , ์ด๊ณผํ๋ ๊ฒฝ์ฐ ๋์คํฌ์ ์ ์ฅ)
- ์ด๋ฏธ์ง, ๋์์, ํฐ ์ด์ง์ฝ๋์ ๊ฐ์ ๋์ฉ๋ ํ์ผ๋ค์ ๋ง์ ๋ฉ๋ชจ๋ฆฌ๋ฅผ ์๋ชจํ์ง ์๊ณ ์ฒ๋ฆฌ ๊ฐ๋ฅ
- ์ ๋ก๋ ๋ ํ์ผ์ ๋ฉํ๋ฐ์ดํฐ๋ฅผ ์ป์ ์ ์์
- file-like async ์ธํฐํ์ด์ค๋ฅผ ๊ฐ๊ณ ์์
- file-like object๋ฅผ ํ์๋กํ๋ ๋ค๋ฅธ ๋ผ์ด๋ธ๋ฌ๋ฆฌ์ ์ง์ ์ ์ผ๋ก ์ ๋ฌํ ์ ์๋ ํ์ด์ฌ SpooledTemporaryFile ๊ฐ์ฒด ๋ฐํ
UploadFile์ ์์ฑ์ ๋ค์๊ณผ ๊ฐ๋ค.
- filename : ๋ฌธ์์ด(str)๋ก ๋ ์ ๋ก๋๋ ํ์ผ์ ํ์ผ๋ช (์: myimage.jpg).
- content_type : ๋ฌธ์์ด(str)๋ก ๋ ํ์ผ ํ์(MIME type / media type)์ด๋ค (์: image/jpeg).
- file : SpooledTemporaryFile (ํ์ผ๋ฅ ๊ฐ์ฒด)์ ๋๋ค. ์ด๊ฒ์ "ํ์ผ๋ฅ" ๊ฐ์ฒด๋ฅผ ํ์๋กํ๋ ๋ค๋ฅธ ๋ผ์ด๋ธ๋ฌ๋ฆฌ์ ์ง์ ์ ์ผ๋ก ์ ๋ฌํ ์ ์๋ ์ค์ง์ ์ธ ํ์ด์ฌ ํ์ผ์ด๋ค.
UploadFile ์๋ ๋ค์์ async ๋ฉ์๋๋ค์ด ์๋ค. ๋ด๋ถ์ ์ธ SpooledTemporaryFile ์ ์ฌ์ฉํ์ฌ ํด๋นํ๋ ํ์ผ ๋ฉ์๋๋ฅผ ํธ์ถํ๋ค.
- write(data): data(str ๋๋ bytes)๋ฅผ ํ์ผ์ ์์ฑํ๋ค.
- read(size): ํ์ผ์ ๋ฐ์ดํธ ๋ฐ ๊ธ์์ size(int)๋ฅผ ์ฝ๋๋ค.
- close(): ํ์ผ์ ๋ซ๋๋ค.
from fastapi import FastAPI, UploadFile
app = FastAPI()
@app.post("/file/")
async def createFile(file: UploadFile):
return {"file": file}

List๋ฅผ ์ฌ์ฉํ์ฌ ์ฌ๋ฌ๊ฐ์ ํ์ผ์ ์ ๋ฌํ ์ ์๋ค.
from typing import List
from fastapi import FastAPI, UploadFile
app = FastAPI()
@app.post("/file/")
async def createFile(files: List[UploadFile]):
return {"files": [f for f in files]}
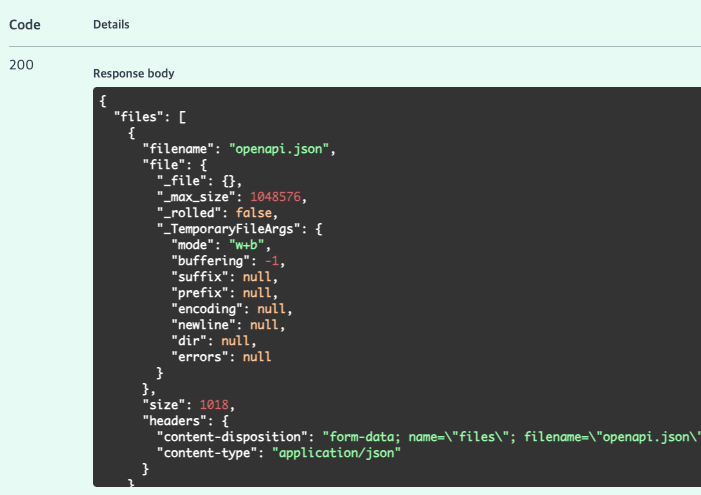
from fastapi import FastAPI, UploadFile
from fastapi.responses import HTMLResponse
import pandas as pd
app = FastAPI()
@app.post("/file/")
async def createFile(file: UploadFile):
contents = await file.read()
df = pd.read_excel(contents)
print(df.head(5))
return {"msg": "success"}
context = """
<body>
<form method="post" action="/file" enctype="multipart/form-data">
file : <input type="file" name="file"><br>
<input type='submit'>
</form>
</body>
"""
@app.get('/')
async def main():
return HTMLResponse(context)
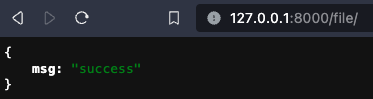
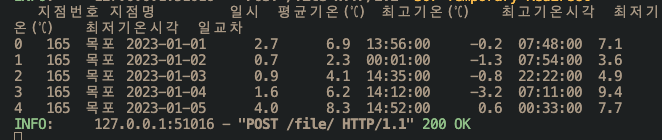
'Back-end & Server > FastAPI' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
[FastAPI] ๋ฏธ๋ค์จ์ด (0) | 2024.02.20 |
---|---|
[FastAPI] ๊ฒฝ๋ก ์๋ ์ค์ (0) | 2024.02.20 |
[FastAPI] ๋ชจ๋ธ (0) | 2024.02.20 |
[FastAPI] ๋งค๊ฐ๋ณ์ (0) | 2024.02.20 |
[FastAPI] ๋์์ฑ๊ณผ async / await (0) | 2024.02.19 |