๐ ๋ณธ ์์ ๋ Window10์ VSCode, Python3.11.0๋ก ์์ฑ๋์์ต๋๋ค.
ํ ์คํธ๋ฅผ ์ฝ์ ํ๋ ๋ฉ์๋๋ "putText"์ด๋ค.
import cv2
im = cv2.imread("test.png")
text ="rabbit"
cv2.putText(
img=im,
text=text,
org=(50,50),
fontFace=cv2.FONT_HERSHEY_SIMPLEX,
fontScale=1,
color=(242,200,100),
thickness=1,
lineType=cv2.LINE_AA,
bottomLeftOrigin=False
)
cv2.imshow("text",im)
cv2.waitKey(0)
cv2.destroyAllWindows()
๋งค๊ฐ๋ณ์๋ ๋ค์๊ณผ ๊ฐ๋ค.
- img : ์ด๋ฏธ์ง
- text : ์ฝ์ ํ ํ ์คํธ
- org : ๋ฌธ์์ด์ ์ถ๋ ฅํ ์์น์ ์ข์ธก ํ๋จ ์ขํ
- fontFace : ํฐํธ
- fontScale : ํฐํธ ์ค์ผ์ผ
- color : ์ปฌ๋ฌ
- thickness : ์ ๊ตต๊ธฐ
- lineType : ์ ํ์
- bottomLeftOrigin : True์ ๊ฒฝ์ฐ ๊ธ์๊ฐ ์์๋๋ก ๋ฐ์ ๋จ
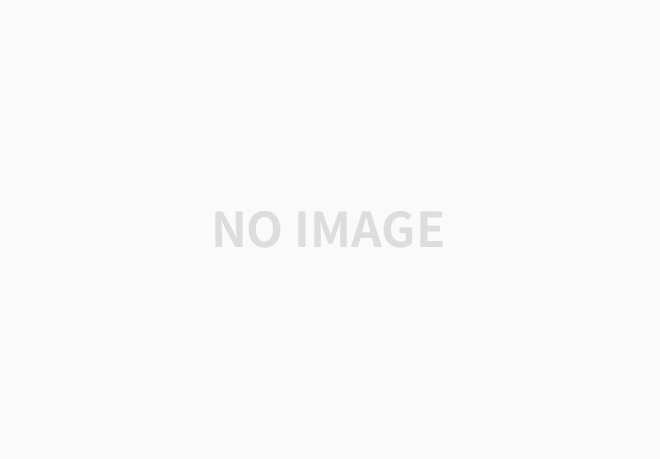
Trackbar๋ผ๋ ๊ธฐ๋ฅ์ ์ฌ์ฉํด ๋์ ์ผ๋ก ์ด๋ฏธ์ง์ ๋ณํ๋ฅผ ์ค ์ ์๋ค.
import cv2
img = cv2.imread("test.png",cv2.IMREAD_GRAYSCALE)
img = cv2.resize(img,(512,512),interpolation=cv2.INTER_LANCZOS4)
def on_change(pos:int):
_,binary = cv2.threshold(img,pos,255,cv2.THRESH_BINARY)
cv2.imshow("thres",binary)
cv2.imshow("thres",img)
cv2.createTrackbar("Threshold Controler","thres",100,125,on_change)
cv2.waitKey(0)
cv2.destroyAllWindows()
createTrackbar์ on_change ํจ์๋ฅผ ๊ฐ์ด ์ฌ์ฉํ๋ฉด ๋์ ์ผ๋ก ์ด๋ฏธ์ง๋ฅผ ์์ ํ๋ฉด์ ๊ฒฐ๊ณผ๋ฅผ ์ค์๊ฐ์ผ๋ก ๋ณผ ์ ์๋ค.
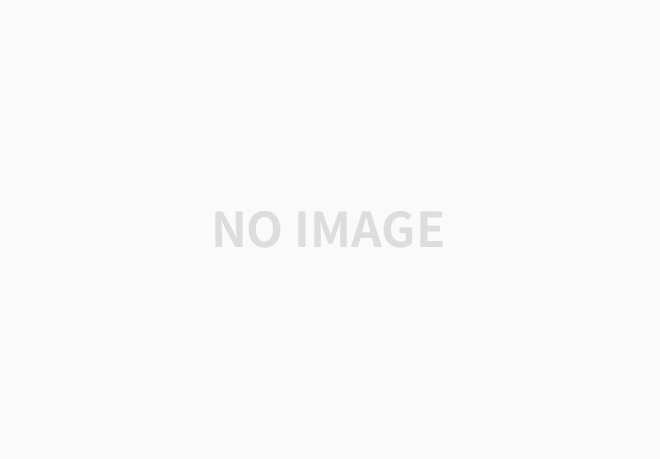
์ ์ฉํ ๊ธฐ๋ฅ์ค ํ๋๋ก ๋ง์คํฌ ์ฐ์ฐ์ด ์๋ค.
Python์์๋ image(3 Channel), mask(2 Channel)๋ฅผ ์ฌ์ฉํด์ ๋ง์คํน ํ ์ ์๋ค.
bitwise ์ฐ์ฐ์ ๋งค๊ฐ๋ณ์๋ ๋ค์๊ณผ ๊ฐ๋ค.
- src1 : ์ฒซ๋ฒ์งธ ์ด๋ฏธ์ง
- src2 : ๋๋ฒ์งธ ์ด๋ฏธ์ง
- dst : ๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ฑฐ๋ ์ถ๋ ฅํ ์ด๋ฏธ์ง, ๊ธฐ๋ณธ๊ฐ None
- mask : ์ ํ ์ฌํญ์ผ๋ก, ํ๋ฐฑ ์ด๋ฏธ์ง์ฌ์ผ ํ๋ฉฐ, ํฐ์ ์์ญ๋ง ์ฐ์ฐ์ ํฌํจ
bitwise_and : ์ผ๋ฐ์ ์ผ๋ก ๋ง์คํน์ ํ ๋ ์ฌ์ฉํจ
import cv2
import numpy as np
img = cv2.imread("test.png")
img = cv2.resize(img,(512,512),interpolation=cv2.INTER_LANCZOS4)
mask = np.zeros(img.shape[:2],dtype=np.uint8)
cv2.rectangle(mask,(100,100),(300,400),255,thickness=cv2.FILLED)
# ๋ง์คํน
inv_mask = cv2.bitwise_and(img,img,mask=mask)
cv2.imshow("inv_mask",inv_mask)
cv2.waitKey(0)
cv2.destroyAllWindows()
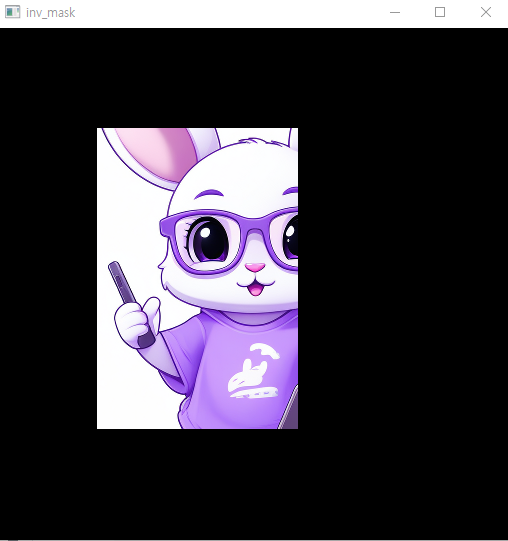
bitwise_not
import cv2
import numpy as np
img = cv2.imread("test.png")
img = cv2.resize(img,(512,512),interpolation=cv2.INTER_LANCZOS4)
mask = np.zeros(img.shape[:2],dtype=np.uint8)
cv2.rectangle(mask,(100,100),(300,400),255,thickness=cv2.FILLED)
# ๋ง์คํน
inv_mask = cv2.bitwise_not(img,img,mask=mask)
cv2.imshow("inv_mask",inv_mask)
cv2.waitKey(0)
cv2.destroyAllWindows()
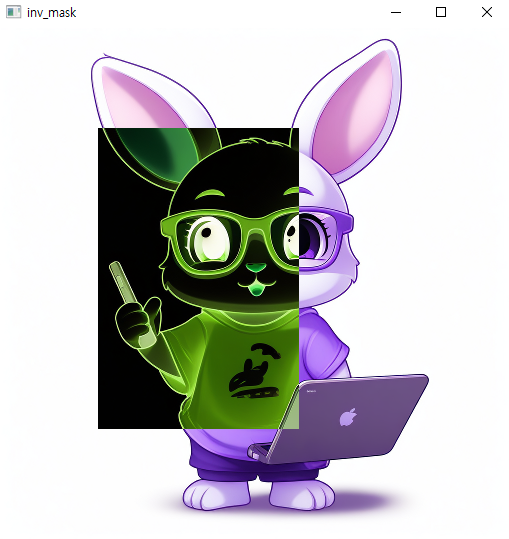
bitwise_or
import cv2
import numpy as np
img = cv2.imread("test.png")
img = cv2.resize(img,(512,512),interpolation=cv2.INTER_LANCZOS4)
mask = np.zeros(img.shape[:2],dtype=np.uint8)
cv2.rectangle(mask,(100,100),(300,400),255,thickness=cv2.FILLED)
# ๋ง์คํน
inv_mask = cv2.bitwise_or(img,img,mask=mask)
cv2.imshow("inv_mask",inv_mask)
cv2.waitKey(0)
cv2.destroyAllWindows()
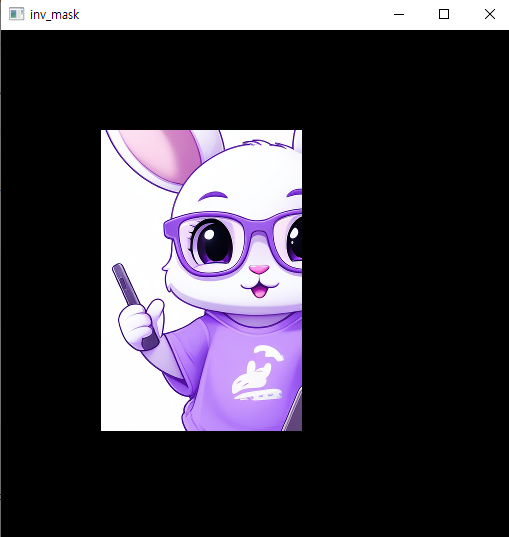
bitwise_xor
import cv2
import numpy as np
img = cv2.imread("test.png")
img = cv2.resize(img,(512,512),interpolation=cv2.INTER_LANCZOS4)
mask = np.zeros(img.shape[:2],dtype=np.uint8)
cv2.rectangle(mask,(100,100),(300,400),255,thickness=cv2.FILLED)
# ๋ง์คํน
inv_mask = cv2.bitwise_xor(img,img,mask=mask)
cv2.imshow("inv_mask",inv_mask)
cv2.waitKey(0)
cv2.destroyAllWindows()
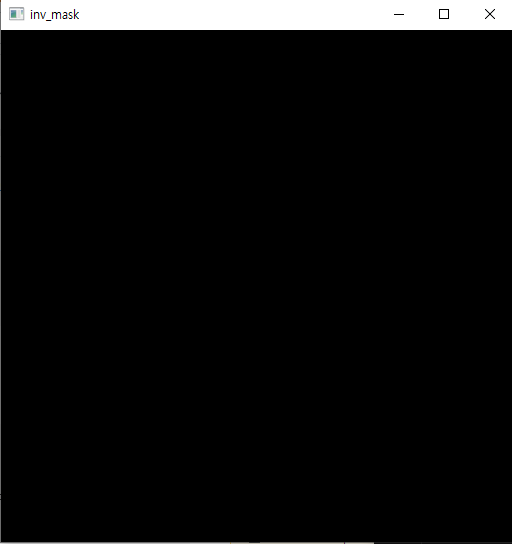
'AI > Computer Vision' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
[Computer Vision] Filtering (0) | 2024.08.30 |
---|---|
[Computer Vision] ์์์ ๋ช ์ ์ ์ด (0) | 2024.08.28 |
[Computer Vision] ๊ทธ๋ฆฌ๊ธฐ ํจ์ (0) | 2024.08.27 |
[Computer Vision] ๊ธฐ๋ณธ ์ฌ์ฉ๋ฒ (0) | 2024.08.27 |
[Computer Vision] Inpainting (0) | 2024.03.25 |