728x90
반응형
IoU(Intersection over Union)는 주로 객체 탐지 및 세그멘테이션 평가에 사용되는 지표이다.
예측한 영역과 실제 영역 간의 겹치는 부분의 비율을 나타낸다.
- x : 영역 중심의 x좌표
- y : 영역 중심의 y좌표
- w : 영역의 폭
- h : 영역의 높이
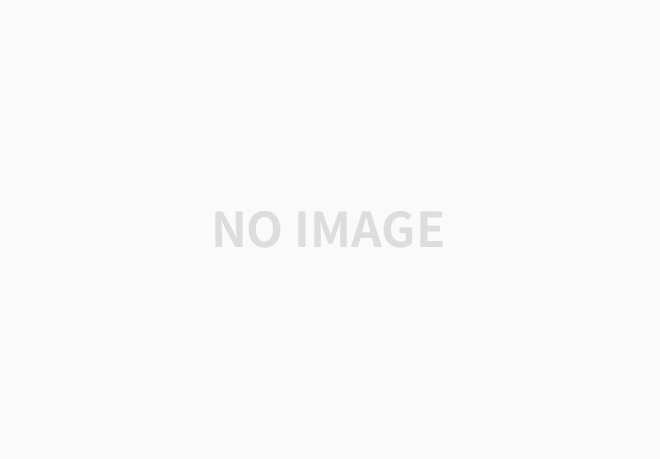
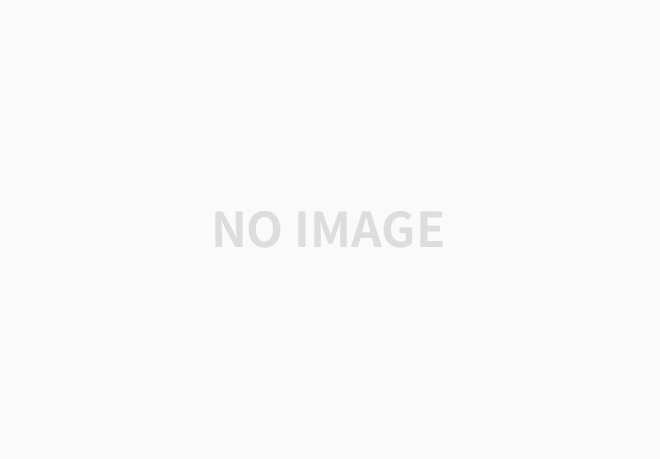
- Area of Intersection : 예측된 영역과 실제 영역의 겹치는 부분의 면적이다.
- Area of Union : 예측된 영역과 실제 영역의 합집합 면적이다.
IoU 값은 0 ~ 1 사이의 값을 가지며, 1에 가까울 수록 예측이 정확하다는 것을 의미한다.
일반적으로 IoU가 0.5 이상이면 긍정적인 예측으로 간주 된다.
import cv2
import numpy as np
def IoU(image:np.ndarray,mask:np.ndarray)->float:
# 마스크를 이진형태로 변환
_,bin_mask = cv2.threshold(mask,127,255,cv2.THRESH_BINARY)
# Intersection과 Union
intersection = np.logical_and(bin_mask,bin_mask).sum()
union = np.logical_or(bin_mask,bin_mask).sum()
# IoU
iou = intersection / (union + 1e-6) # zero division을 방지하기 위해 작은 값 추가
return iou
img = cv2.imread("cat.png")
h,w,_ = img.shape
img = cv2.resize(img,(int(w//2),int(h//2)),interpolation=cv2.INTER_LANCZOS4)
mask_im = cv2.imread("cat_mask.png")
mask_im = cv2.resize(mask_im,(int(w//2),int(h//2)),interpolation=cv2.INTER_LANCZOS4)
mask = cv2.cvtColor(mask_im,cv2.COLOR_BGR2GRAY)
mask[mask!=255] = 0
mask = ~mask
print(IoU(img,mask))
cv2.imshow("original",img)
cv2.imshow("mask_im",mask_im)
cv2.imshow("mask",mask)
cv2.waitKey(0)
cv2.destroyAllWindows()
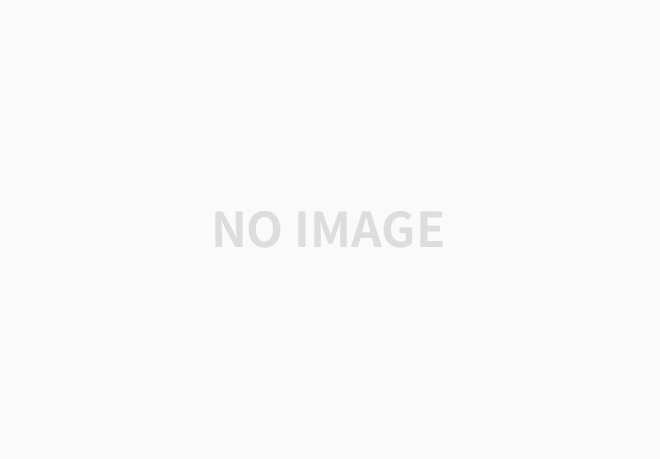
728x90
반응형
'AI > Computer Vision' 카테고리의 다른 글
[Computer Vision] Depth (0) | 2024.09.15 |
---|---|
[Computer Vision] Trapped-ball Segmentation (0) | 2024.09.14 |
[Computer Vision] PIL(Python Image Library) (0) | 2024.09.08 |
[Computer Vision] 지역 특징점 검출과 매칭 (0) | 2024.09.07 |
[Computer Vision] 객체 검출과 응용 (0) | 2024.09.06 |