π λ³Έ μμ λ Window10μ VSCode, Python3.11.0λ‘ μμ±λμμ΅λλ€.
μμμ κΈ°ννμ (Geometric Transform)μ μμμ ꡬμ±νλ ν½μ μ λ°°μΉ κ΅¬μ‘°λ₯Ό λ³κ²½ν¨μΌλ‘μ¨ μ 체 μμμ λͺ¨μμ λ°κΎΈλ μμ μ΄λ€.
μ΄μ νν°λ§μ ν½μ μ μμΉλ₯Ό κ³ μ νκ³ ν½μ μ κ°μ λ³κ²½νμμ§λ§ κΈ°ννμ λ³νμ ν½μ κ°μ κ·Έλλ‘ μ μ§νλ©΄μ μμΉλ₯Ό λ³κ²½νλ μμ μ΄λ€.
Affine Transform
μμμ νν μ΄λμν€κ±°λ νμ , ν¬κΈ° λ³ν λ±μ ν΅ν΄ λ§λ€ μ μλ λ³νμ ν΅μΉνλ€.
Affine λ³νμ κ²½μ° μ§μ κ°μ κΈΈμ΄ λΉμ¨κ³Ό νν κ΄κ³κ° κ·Έλλ‘ μ μ§λλ€.
# transforms.py
import cv2
import numpy as np
def affineTransform(image:np.ndarray,matrix:np.ndarray,shape:tuple):
return cv2.warpAffine(image,matrix,shape)
# main.py
import cv2
import numpy as np
# affine
from transforms import affineTransform
def show(origin:np.ndarray,result:np.ndarray):
cv2.imshow("origin",origin)
cv2.imshow("result",result)
cv2.waitKey(0)
cv2.destroyAllWindows()
img = cv2.imread("test.png")
img = cv2.resize(img,(480,480))
points_src = np.float32([[50, 50], [200, 50], [50, 200]]) # λ³ν μ μ μ λ€
points_dst = np.float32([[10, 100], [200, 50], [100, 250]]) # λ³ν νμ μ λ€
matrix = cv2.getAffineTransform(points_src,points_dst)
aff_result = affineTransform(img,matrix,img.shape[:2])
show(img,aff_result)
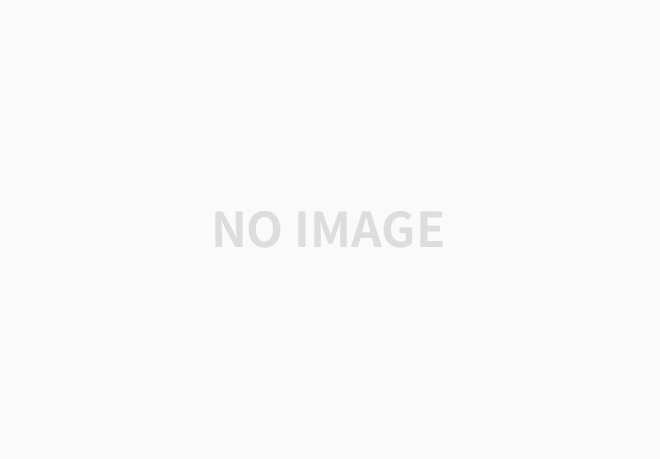
Translation Transformation
μ΄λ λ³νμ μμμ κ°λ‘ λλ μΈλ‘ λ°©ν₯μΌλ‘ μΌμ ν¬κΈ°λ§νΌ μ΄λμν€λ μ°μ°(μννΈ μ°μ°)μ μλ―Ένλ€.
# transforms.py
import cv2
import numpy as np
def translationTransform(image:np.ndarray,matrix:np.ndarray,shape:tuple):
return cv2.warpAffine(image,matrix,shape)
# main.py
import cv2
import numpy as np
# affine
from transforms import affineTransform
def show(origin:np.ndarray,result:np.ndarray):
cv2.imshow("origin",origin)
cv2.imshow("result",result)
cv2.waitKey(0)
cv2.destroyAllWindows()
img = cv2.imread("test.png")
img = cv2.resize(img,(480,480))
tx = 100 # xμΆμΌλ‘ μ΄λν 거리
ty = 50 # yμΆμΌλ‘ μ΄λν 거리
matrix = np.float32([[1,0,tx],[0,1,ty]])
result = translationTransform(img,matrix,img.shape[:2])
show(img,result)
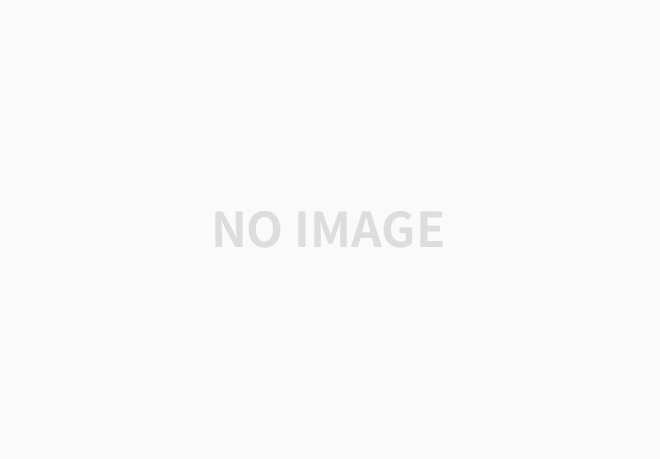
Shear Transformation
μ λ¨ λ³νμ μ§μ¬κ°ν ννμ μμμ νμͺ½ λ°©ν₯μ λ°μ΄μ ννμ¬λ³ν λͺ¨μμΌλ‘ λ³νλλ λ³νμ΄λ©° μΈ΅λ°λ¦Ό λ³νμ΄λΌκ³ λ νλ€.
# transforms.py
import cv2
import numpy as np
def transformation(image:np.ndarray,matrix:np.ndarray,shape:tuple):
return cv2.warpAffine(image,matrix,shape)
# main.py
import cv2
import numpy as np
# affine
from transforms import transformation
def show(origin:np.ndarray,result:np.ndarray):
cv2.imshow("origin",origin)
cv2.imshow("result",result)
cv2.waitKey(0)
cv2.destroyAllWindows()
img = cv2.imread("test.png")
img = cv2.resize(img,(480,480))
shear_factor = 0.5
matrix = np.float32([[1, shear_factor, 0], [0, 1, 0]])
shape = img.shape[:2]
result = transformation(img,matrix,(int(shape[0]+shape[1] * shear_factor),shape[1]))
show(img,result)
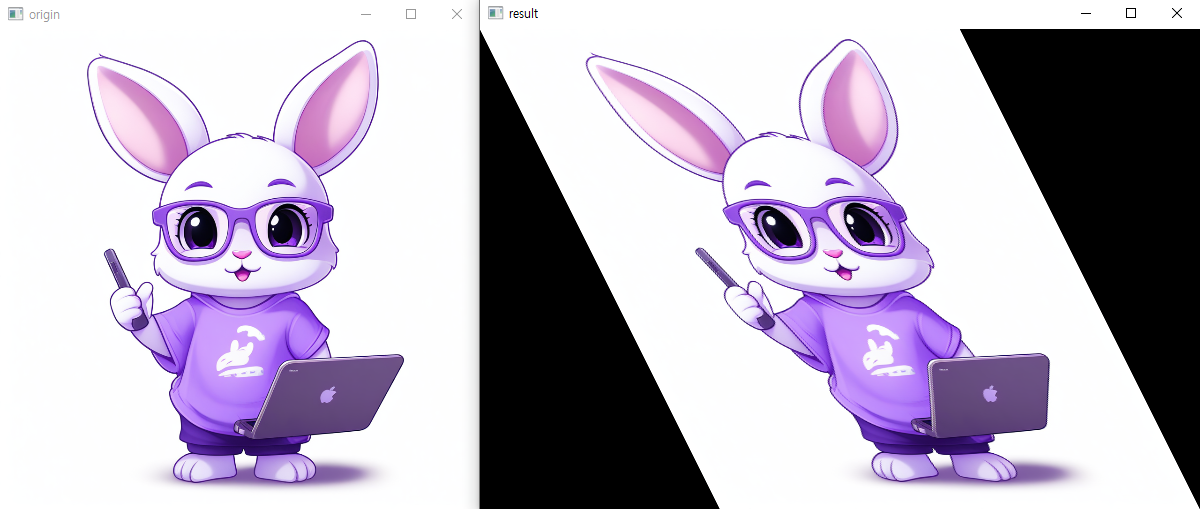
Scale Transformation
μμμ ν¬κΈ° λ³νμ μμμ μ 체μ μΈ ν¬κΈ°λ₯Ό νλ λλ μΆμνλ λ³νμ΄λ€.
μμμ μ€μΌμΌλ§ μ μμ μμ€μ΄ μκΈ° λλ¬Έμ κ·Έλμ λ§λ 보κ°λ²μΌλ‘ μμ μμ€μ μ΅μν ν΄μΌνλ€.
InterpolationFlags
- cv2.INTER_NEAREST : κ°μ₯ κ°λ¨, κ°μ₯ κ°κΉμ΄ ν½μ κ°μ μ¬μ©
- cv2. INTER_LINEAR : 2x2 ν½μ λ€νΈμν¬μμ μ ν λ³΄κ° μν, μΌλ°μ μΈ νλ λ° μΆμμ μ ν©
- cv2. INTER_CUBIC : 4x4 ν½μ λ€νΈμν¬μμ 3μ°¨ λ³΄κ° μ¬μ©, νλν λ μ£Όλ‘ μ¬μ©
- cv2. INTER_AREA : ν½μ μμ νκ· μ μ¬μ©νμ¬ μ΄λ―Έμ§λ₯Ό μΆμν λ μ£Όλ‘ μ¬μ©, μΆμ μ νμ§ μ’κ³ , λͺ¨μμ΄ν¬ ν¨κ³Όλ₯Ό μ€μ΄λ λ° ν¨κ³Όμ
- cv2. INTER_LANCZOS4 : 8x8 ν½μ λ€νΈμν¬μμ Lanczos μ¬μνλ§μ μ¬μ©, κ°μ₯ μ±λ₯μ΄ μ’κ³ μΆμμ λ ν¨κ³Όμ
import cv2
import numpy as np
def show(origin:np.ndarray,result:np.ndarray):
cv2.imshow("origin",origin)
cv2.imshow("result",result)
cv2.waitKey(0)
cv2.destroyAllWindows()
img = cv2.imread("test.png")
img = cv2.resize(img,(480,480))
result = cv2.resize(img,None,fx=1.5,fy=1.5,interpolation=cv2.INTER_LANCZOS4)
show(img,result)
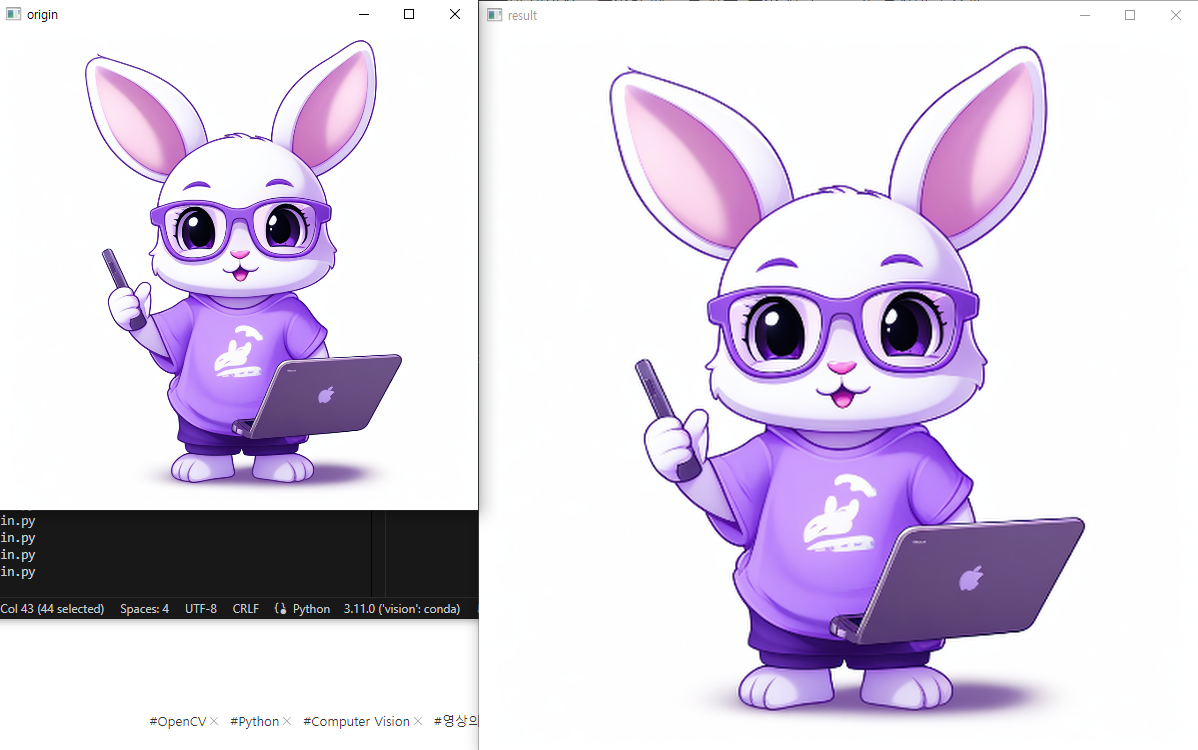
Rotation Transformation
μμ μ²λ¦¬ μμ€ν μ λ§λ€λ€λ³΄λ©΄ μμμ νμ μμΌμΌν λκ° μ’ μ’ λ°μνλ€.
μ΄λ μ¬μ©νλ νμ λ³νμ νΉμ μ’νλ₯Ό κΈ°μ€μΌλ‘ μμμ μνλ κ°λλ§νΌ νμ νλ λ³νμ΄λ€.
import cv2
import numpy as np
# affine
from transforms import transformation
def show(origin:np.ndarray,result:np.ndarray):
cv2.imshow("origin",origin)
cv2.imshow("result",result)
cv2.waitKey(0)
cv2.destroyAllWindows()
img = cv2.imread("test.png")
img = cv2.resize(img,(480,480))
# νμ μΆ μ’ν
h,w,_ = img.shape
center = (h // 2,w // 2)
# νμ κ°λ
angle = 45
# λ³νΈλ νλ ¬
matrix = cv2.getRotationMatrix2D(center,angle,1.0) # λ§μ§λ§ μΈμλ μ€μΌμΌλ§
result = transformation(img,matrix,(w,h))
show(img,result)
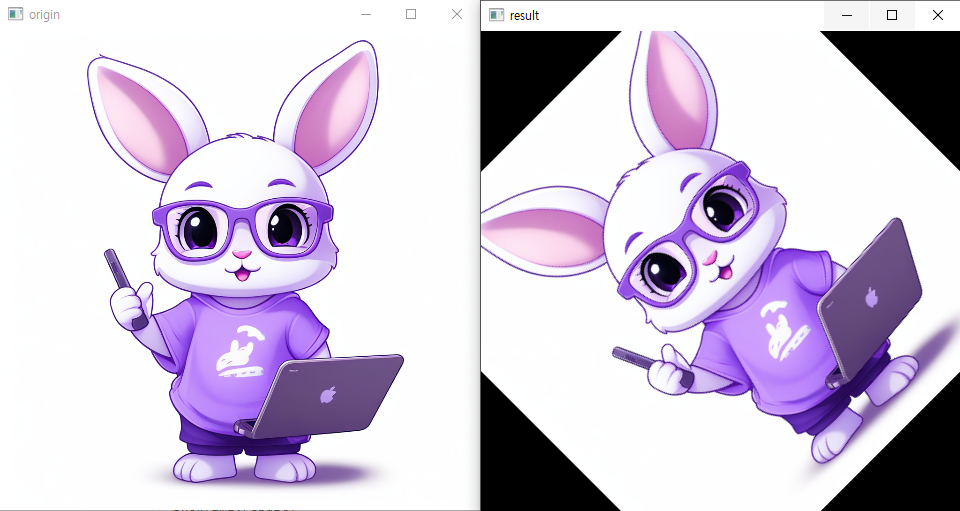
Symmetry Transformation
λμΉ λ³νμ μμμ λ§μΉ κ±°μΈμ λΉμΉ κ²μ²λΌ μ’μ°λ₯Ό λ°κΎΈλ λ³νμ μ’μ° λμΉ λλ μ’μ° λ°μ μ΄λΌκ³ νλ€.
μ λ ₯ μμκ³Ό μΆλ ₯ μμμ΄ μΌλμΌ λμλλ―λ‘ λ³΄κ°λ²μ΄ νμνμ§ μλλ€.
import cv2
import numpy as np
def show(origin:np.ndarray,result:np.ndarray):
cv2.imshow("origin",origin)
cv2.imshow("result",result)
cv2.waitKey(0)
cv2.destroyAllWindows()
img = cv2.imread("test.png")
img = cv2.resize(img,(480,480))
cv2.imshow("origin",origin)
# μν λμΉ (XμΆ κΈ°μ€)
horizontal_flip = cv2.flip(img, 0)
cv2.imshow("horizon",horizontal_flip)
# μμ§ λμΉ (YμΆ κΈ°μ€)
vertical_flip = cv2.flip(img, 1)
cv2.imshow("vertical",vertical_flip)
# μ€μ¬ λμΉ (μμ κΈ°μ€)
both_flip = cv2.flip(img, -1)
cv2.imshow("both",both_flip)
cv2.waitKey(0)
cv2.destroyAllWindows()
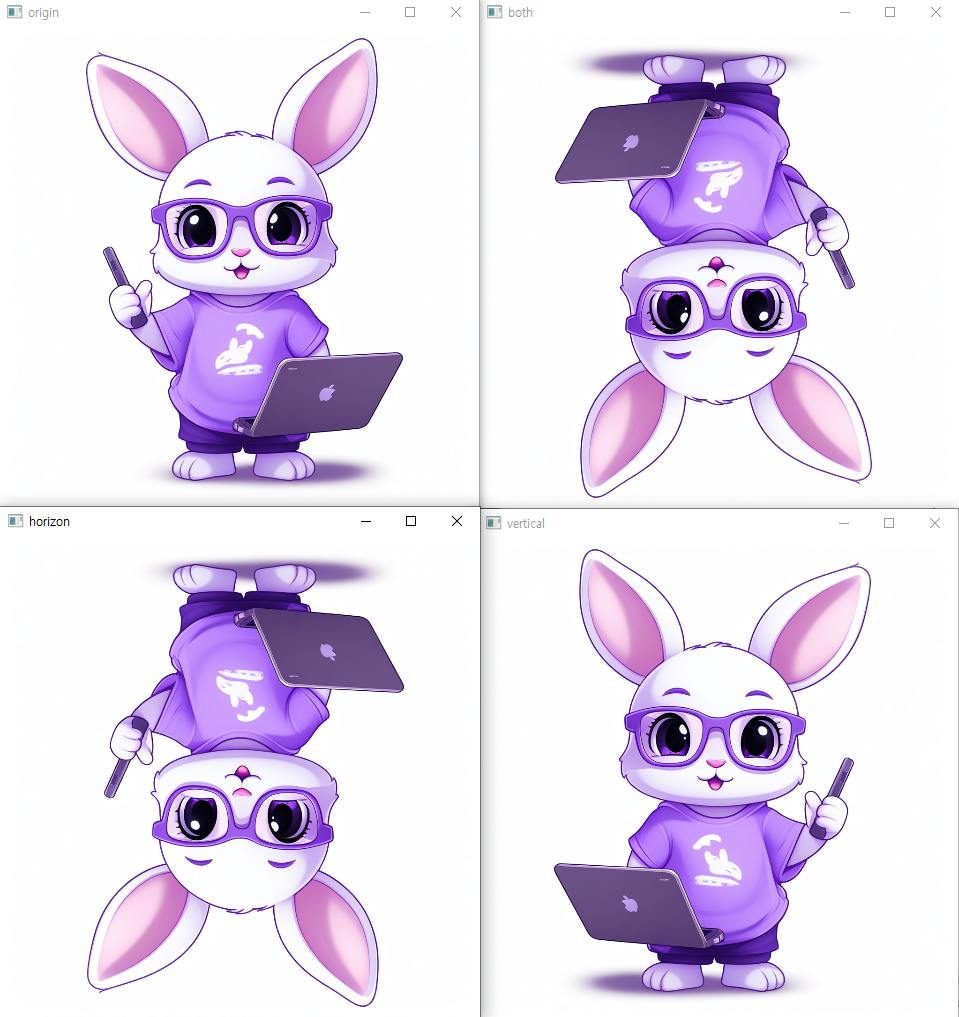
Perspectuve Transform
ν¬μ λ³νμ μ§μ¬κ°ν ννμ μμμ μμμ λ³Όλ‘ μ¬κ°ν ννλ‘ λ³κ²½ν μ μλ λ³νμ΄λ€.
ν¬μ λ³νμ μν΄ μλ³Έ μμμ μλ μ§μ μ κ²°κ³Ό μμμμ κ·Έλλ‘ μ§μ μ±μ΄ μ μ§λμ§λ§, λ μ§μ μ νν κ΄κ³λ κΉ¨μ΄μ§ μ μλ€.
import cv2
import numpy as np
# affine
from transforms import transformation_per
def show(origin:np.ndarray,result:np.ndarray):
cv2.imshow("origin",origin)
cv2.imshow("result",result)
cv2.waitKey(0)
cv2.destroyAllWindows()
img = cv2.imread("test.png")
img = cv2.resize(img,(480,480))
# μλ³Έ μ΄λ―Έμ§μμ λ³νν λ€ μ (μμμ )
points = np.float32([[50, 50], [200, 50], [50, 200], [200, 200]])
# λ³νν λ€ μ (λμμ )
dst_points = np.float32([[10, 10], [300, 50], [50, 250], [250, 200]])
# ν¬μ λ³ν νλ ¬ μμ±
matrix = cv2.getPerspectiveTransform(points, dst_points)
# μ΄λ―Έμ§μ ν¬μ λ³ν μ μ©
height, width = img.shape[:2]
result = cv2.warpPerspective(img, matrix, (width, height))
show(img,result)
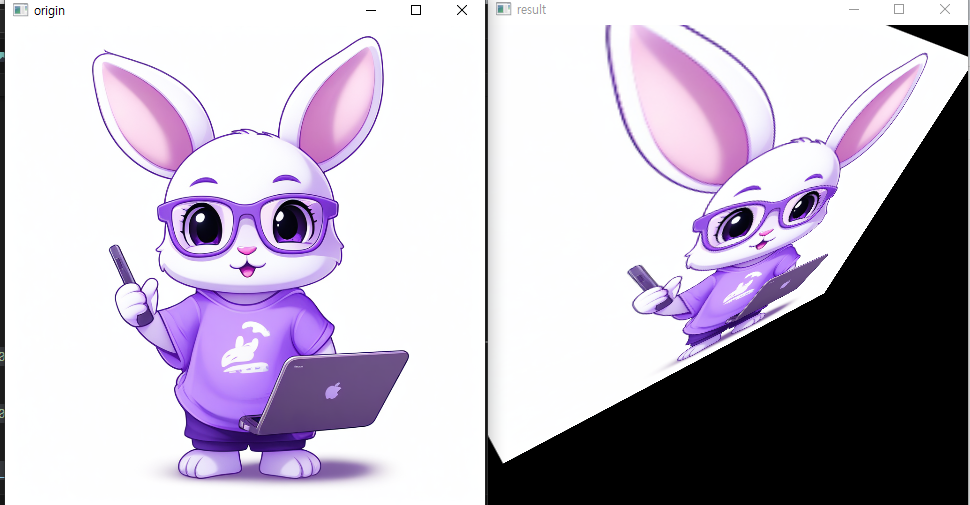
'AI > Computer Vision' μΉ΄ν κ³ λ¦¬μ λ€λ₯Έ κΈ
[Computer Vision] μ»¬λ¬ μμ μ²λ¦¬ (0) | 2024.09.02 |
---|---|
[Computer Vision] μμ§(Edge) κ²μΆ (0) | 2024.09.02 |
[Computer Vision] Filtering (0) | 2024.08.30 |
[Computer Vision] μμμ λͺ μ μ μ΄ (0) | 2024.08.28 |
[Computer Vision] ν μ€νΈ μ½μ λ° μ μ©ν κΈ°λ₯ (0) | 2024.08.28 |